animated gifs
ebay html animated gifs
JAVA APPLET
http://www.bellsnwhistles.com/bells03.html
Liquid Metal Applet
=========
==========
file:///C:/DOCUME~1/USER/LOCALS~1/Temp/Rar$EX45.485/SpaceFlight.html
SpaceFlight
http://javaboutique.internet.com/SpaceFlight/
CODE="SpaceFlight.class"
WIDTH="400"
HEIGHT="344">
.............
Java Source:
import java.awt.event.*;
import java.awt.*;
import java.io.Serializable;
import java.applet.*;
import java.util.*;
class Fly_by_Star {
int Xmax, Ymax, z;
double x, y;
Fly_by_Star( int width, int height )
{
Xmax = width/2;
Ymax = height/2;
x = (Math.random()*width) - Xmax;
y = (Math.random()*height) - Ymax;
if( (x == 0) && (y == 0 ) ) x = 10;
z = (int)(Math.random()*100);
}
public void Draw( Graphics g, int mx, int my, int dx, int dy, double crot, double srot )
{
double X, Y;
int h, v;
int d;
z-=2;
x = x - ((double)mx*crot/25 -(double)my*srot/25)*(z+62)/162;
y = y + ((double)mx*srot/25 -(double)my*crot/25)*(z+62)/162;
if (x<-Xmax)x+=2*Xmax;
if (x>Xmax)x-=2*Xmax;
if (y<-Ymax)y+=2*Ymax;
if (y>Ymax)y-=2*Ymax;
X = (x*128/(64+z));
Y = (y*128/(64+z));
if( (X < -Xmax) || (X > Xmax)) z = 100;
if( (Y < -Ymax) || (Y > Xmax)) z = 100;
h = (int)(X*crot-Y*srot)+Xmax;
v = (int)(X*srot+Y*crot)+Ymax;
if ( z > 50 )g.setColor( Color.gray );
else if ( z > 25 )g.setColor( Color.lightGray );
else g.setColor( Color.white );
d=(100-z)/40;
if( d == 0 ) d = 1;
if( d == 1 ) g.setColor( Color.white );
g.fillRect( h, v, d, d );
}
}
class Background_Star {
int Xmax, Ymax, z;
double x, y;
Background_Star( int width, int height )
{
Xmax = width/2;
Ymax = height/2;
x = (Math.random()*width) - Xmax;
y = (Math.random()*height) - Ymax;
if( (x == 0) && (y == 0 ) ) x = 10;
z = (int)(Math.random()*100); /* Here it means brightness */
}
public void BDraw( Graphics g, int mx, int my, int dx, int dy, double crot, double srot)
{
int h, v;
int d;
x = x - (double)mx*crot/25 - (double)my*srot/25;
y = y + (double)mx*srot/25 - (double)my*crot/25;
if (x<-Xmax)x+=2*Xmax;
if (x>Xmax)x-=2*Xmax;
if (y<-Ymax)y+=2*Ymax;
if (y>Ymax)y-=2*Ymax;
h = (int)(x*crot-y*srot)+Xmax;
v = (int)(x*srot+y*crot)+Ymax;
if (h<0)h+=2*Xmax;
if (h>2*Xmax)h-=2*Xmax;
if (v<0)v+=2*Ymax;
if (v>2*Ymax)v-=2*Ymax;
if ( z > 50 )g.setColor( Color.gray );
else if( z > 25 )g.setColor( Color.lightGray );
else g.setColor( Color.lightGray );
d=(100-z)/50;
if( d == 0 ) d = 1;
g.fillRect( h, v, d, d );
}
}
public class SpaceFlight extends Applet implements Runnable, MouseMotionListener, MouseListener, Serializable
{
int Width, Height, Xmax, Ymax;
Thread me = null;
boolean suspend = false;
Image im;
Graphics offscreen;
Cursor fifi;
int speed, N_Stars;
int mx, my, dx, dy, mxold, myold; /* m: mouse pixel from center */
double crot, srot, rot;
Fly_by_Star FStar[]; /* Fly-by Star */
Background_Star BStar[]; /* Background */
public void init()
{
Width = getSize().width;
Height = getSize().height;
String Velocity = getParameter( "Velocity" );
Show( "speed", Velocity );
speed = (Velocity == null ) ? 50 : Integer.valueOf( Velocity ).intValue();
String Number_of_Stars = getParameter( "Number_of_Stars" );
Show( "Number_of_Stars", Number_of_Stars );
N_Stars = (Number_of_Stars == null ) ? 30 : Integer.valueOf( Number_of_Stars ).intValue();
try
{
im = createImage( Width, Height );
offscreen = im.getGraphics();
}
catch( Exception e)
{
offscreen = null;
}
BStar = new Background_Star[N_Stars];
FStar = new Fly_by_Star[N_Stars];
for( int i = 0; i < N_Stars; i++ )
{
BStar[i] = new Background_Star( Width, Height );
FStar[i] = new Fly_by_Star( Width, Height );
}
mxold = 0;
myold = 0;
Xmax = Width/2;
Ymax = Height/2;
addMouseMotionListener(this);
addMouseListener(this);
}
public void paint( Graphics g )
{
if( offscreen != null )
{
paintMe( offscreen );
g.drawImage( im, 0, 0, this );
}
else
{
paintMe( g );
}
}
public void paintMe( Graphics g )
{
g.setColor( Color.black );
g.fillRect( 0, 0, Width, Height );
for( int i = 0; i < N_Stars; i++ )
{
BStar[i].BDraw( g, mx, my, dx, dy, crot, srot );
FStar[i].Draw( g, mx, my, dx, dy, crot, srot );
}
}
public void start()
{
getFrame(this).setCursor(Frame.CROSSHAIR_CURSOR) ;
if( me == null )
{
me = new Thread( this );
me.start();
}
}
public void stop()
{
if( me != null )
{
me.stop();
me = null;
}
getFrame(this).setCursor(Frame.DEFAULT_CURSOR) ;
}
public void run()
{
while( me != null )
{
dx = mx - mxold;
dy = my - myold;
mxold = mx;
myold = my;
rot = 3.14/6*mx/Xmax*0; /*disabled*/
crot = Math.cos(rot);
srot = Math.sin(rot);
try { Thread.sleep( speed ); }
catch (InterruptedException e){}
repaint();
}
}
public void update( Graphics g )
{
paint( g );
}
public Frame getFrame(Component c) { while( c != null && !(c instanceof java.awt.Frame) ) c = c.getParent(); return (Frame) c; }
public void mouseMoved(MouseEvent evt)
{
mx = evt.getX()-Xmax;
my = evt.getY()-Ymax;
}
public void mouseDragged(MouseEvent evt) {}
public void mouseReleased(MouseEvent e) { }
public void mousePressed(MouseEvent e) { }
public void mouseClicked(MouseEvent e) { }
public void mouseEntered(MouseEvent e) { }
public void mouseExited(MouseEvent e) { }
public void Toggle( )
{
if( me != null )
{
if( suspend )
{
me.resume();
}
else
{
me.suspend();
}
suspend = !suspend;
}
}
public void Show( String theString, String theValue )
{
if( theValue == null )
{
System.out.println( theString + " : null");
}
else
{
System.out.println( theString + " : " + theValue );
}
}
}
=================================================================
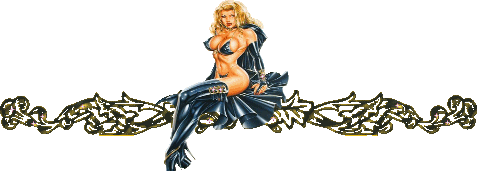
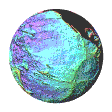
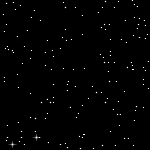

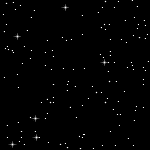


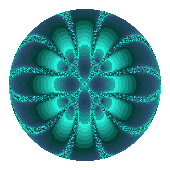


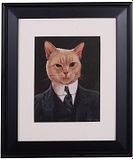
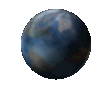
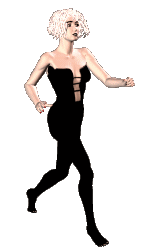
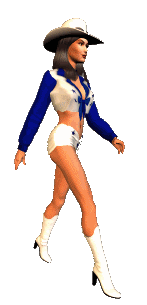
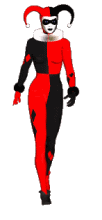
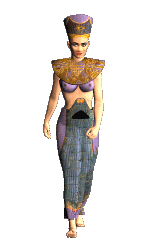
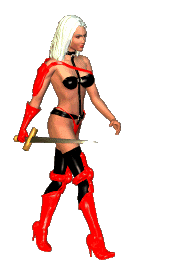
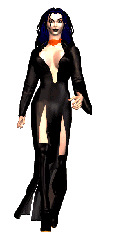









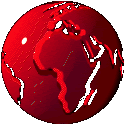

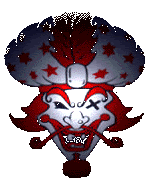



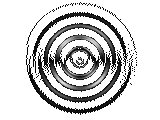

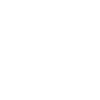


JAVA APPLET
http://www.bellsnwhistles.com/bells03.html
Liquid Metal Applet
=========
==========
file:///C:/DOCUME~1/USER/LOCALS~1/Temp/Rar$EX45.485/SpaceFlight.html
SpaceFlight
http://javaboutique.internet.com/SpaceFlight/
CODE="SpaceFlight.class"
WIDTH="400"
HEIGHT="344">
.............
Java Source:
import java.awt.event.*;
import java.awt.*;
import java.io.Serializable;
import java.applet.*;
import java.util.*;
class Fly_by_Star {
int Xmax, Ymax, z;
double x, y;
Fly_by_Star( int width, int height )
{
Xmax = width/2;
Ymax = height/2;
x = (Math.random()*width) - Xmax;
y = (Math.random()*height) - Ymax;
if( (x == 0) && (y == 0 ) ) x = 10;
z = (int)(Math.random()*100);
}
public void Draw( Graphics g, int mx, int my, int dx, int dy, double crot, double srot )
{
double X, Y;
int h, v;
int d;
z-=2;
x = x - ((double)mx*crot/25 -(double)my*srot/25)*(z+62)/162;
y = y + ((double)mx*srot/25 -(double)my*crot/25)*(z+62)/162;
if (x<-Xmax)x+=2*Xmax;
if (x>Xmax)x-=2*Xmax;
if (y<-Ymax)y+=2*Ymax;
if (y>Ymax)y-=2*Ymax;
X = (x*128/(64+z));
Y = (y*128/(64+z));
if( (X < -Xmax) || (X > Xmax)) z = 100;
if( (Y < -Ymax) || (Y > Xmax)) z = 100;
h = (int)(X*crot-Y*srot)+Xmax;
v = (int)(X*srot+Y*crot)+Ymax;
if ( z > 50 )g.setColor( Color.gray );
else if ( z > 25 )g.setColor( Color.lightGray );
else g.setColor( Color.white );
d=(100-z)/40;
if( d == 0 ) d = 1;
if( d == 1 ) g.setColor( Color.white );
g.fillRect( h, v, d, d );
}
}
class Background_Star {
int Xmax, Ymax, z;
double x, y;
Background_Star( int width, int height )
{
Xmax = width/2;
Ymax = height/2;
x = (Math.random()*width) - Xmax;
y = (Math.random()*height) - Ymax;
if( (x == 0) && (y == 0 ) ) x = 10;
z = (int)(Math.random()*100); /* Here it means brightness */
}
public void BDraw( Graphics g, int mx, int my, int dx, int dy, double crot, double srot)
{
int h, v;
int d;
x = x - (double)mx*crot/25 - (double)my*srot/25;
y = y + (double)mx*srot/25 - (double)my*crot/25;
if (x<-Xmax)x+=2*Xmax;
if (x>Xmax)x-=2*Xmax;
if (y<-Ymax)y+=2*Ymax;
if (y>Ymax)y-=2*Ymax;
h = (int)(x*crot-y*srot)+Xmax;
v = (int)(x*srot+y*crot)+Ymax;
if (h<0)h+=2*Xmax;
if (h>2*Xmax)h-=2*Xmax;
if (v<0)v+=2*Ymax;
if (v>2*Ymax)v-=2*Ymax;
if ( z > 50 )g.setColor( Color.gray );
else if( z > 25 )g.setColor( Color.lightGray );
else g.setColor( Color.lightGray );
d=(100-z)/50;
if( d == 0 ) d = 1;
g.fillRect( h, v, d, d );
}
}
public class SpaceFlight extends Applet implements Runnable, MouseMotionListener, MouseListener, Serializable
{
int Width, Height, Xmax, Ymax;
Thread me = null;
boolean suspend = false;
Image im;
Graphics offscreen;
Cursor fifi;
int speed, N_Stars;
int mx, my, dx, dy, mxold, myold; /* m: mouse pixel from center */
double crot, srot, rot;
Fly_by_Star FStar[]; /* Fly-by Star */
Background_Star BStar[]; /* Background */
public void init()
{
Width = getSize().width;
Height = getSize().height;
String Velocity = getParameter( "Velocity" );
Show( "speed", Velocity );
speed = (Velocity == null ) ? 50 : Integer.valueOf( Velocity ).intValue();
String Number_of_Stars = getParameter( "Number_of_Stars" );
Show( "Number_of_Stars", Number_of_Stars );
N_Stars = (Number_of_Stars == null ) ? 30 : Integer.valueOf( Number_of_Stars ).intValue();
try
{
im = createImage( Width, Height );
offscreen = im.getGraphics();
}
catch( Exception e)
{
offscreen = null;
}
BStar = new Background_Star[N_Stars];
FStar = new Fly_by_Star[N_Stars];
for( int i = 0; i < N_Stars; i++ )
{
BStar[i] = new Background_Star( Width, Height );
FStar[i] = new Fly_by_Star( Width, Height );
}
mxold = 0;
myold = 0;
Xmax = Width/2;
Ymax = Height/2;
addMouseMotionListener(this);
addMouseListener(this);
}
public void paint( Graphics g )
{
if( offscreen != null )
{
paintMe( offscreen );
g.drawImage( im, 0, 0, this );
}
else
{
paintMe( g );
}
}
public void paintMe( Graphics g )
{
g.setColor( Color.black );
g.fillRect( 0, 0, Width, Height );
for( int i = 0; i < N_Stars; i++ )
{
BStar[i].BDraw( g, mx, my, dx, dy, crot, srot );
FStar[i].Draw( g, mx, my, dx, dy, crot, srot );
}
}
public void start()
{
getFrame(this).setCursor(Frame.CROSSHAIR_CURSOR) ;
if( me == null )
{
me = new Thread( this );
me.start();
}
}
public void stop()
{
if( me != null )
{
me.stop();
me = null;
}
getFrame(this).setCursor(Frame.DEFAULT_CURSOR) ;
}
public void run()
{
while( me != null )
{
dx = mx - mxold;
dy = my - myold;
mxold = mx;
myold = my;
rot = 3.14/6*mx/Xmax*0; /*disabled*/
crot = Math.cos(rot);
srot = Math.sin(rot);
try { Thread.sleep( speed ); }
catch (InterruptedException e){}
repaint();
}
}
public void update( Graphics g )
{
paint( g );
}
public Frame getFrame(Component c) { while( c != null && !(c instanceof java.awt.Frame) ) c = c.getParent(); return (Frame) c; }
public void mouseMoved(MouseEvent evt)
{
mx = evt.getX()-Xmax;
my = evt.getY()-Ymax;
}
public void mouseDragged(MouseEvent evt) {}
public void mouseReleased(MouseEvent e) { }
public void mousePressed(MouseEvent e) { }
public void mouseClicked(MouseEvent e) { }
public void mouseEntered(MouseEvent e) { }
public void mouseExited(MouseEvent e) { }
public void Toggle( )
{
if( me != null )
{
if( suspend )
{
me.resume();
}
else
{
me.suspend();
}
suspend = !suspend;
}
}
public void Show( String theString, String theValue )
{
if( theValue == null )
{
System.out.println( theString + " : null");
}
else
{
System.out.println( theString + " : " + theValue );
}
}
}
=================================================================
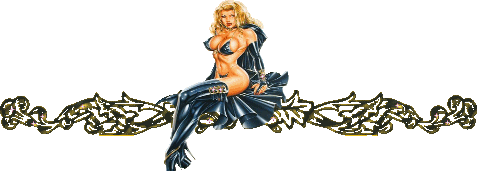
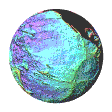
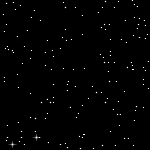

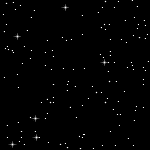


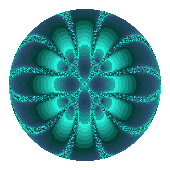


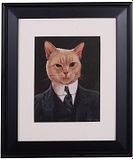
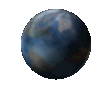
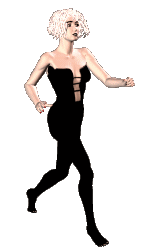
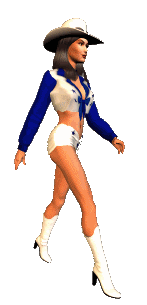
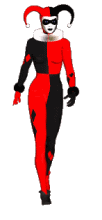
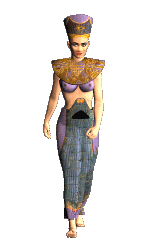
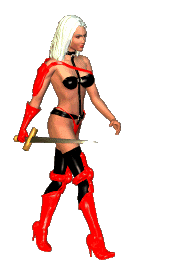
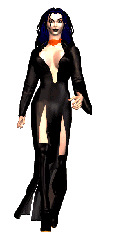









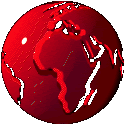

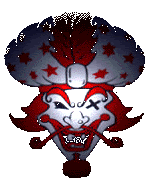



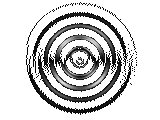

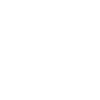


0 Comments:
Post a Comment
<< Home